【C言語】第3章第5回:文字列操作関数:strcpy, strcat, strlenなど
C言語の文字列操作には、strcpy
、strcat
、strlen
など、標準ライブラリ関数が便利です。この章では、それらの関数の使い方を詳しく解説します。
1. strcpy関数:文字列のコピー
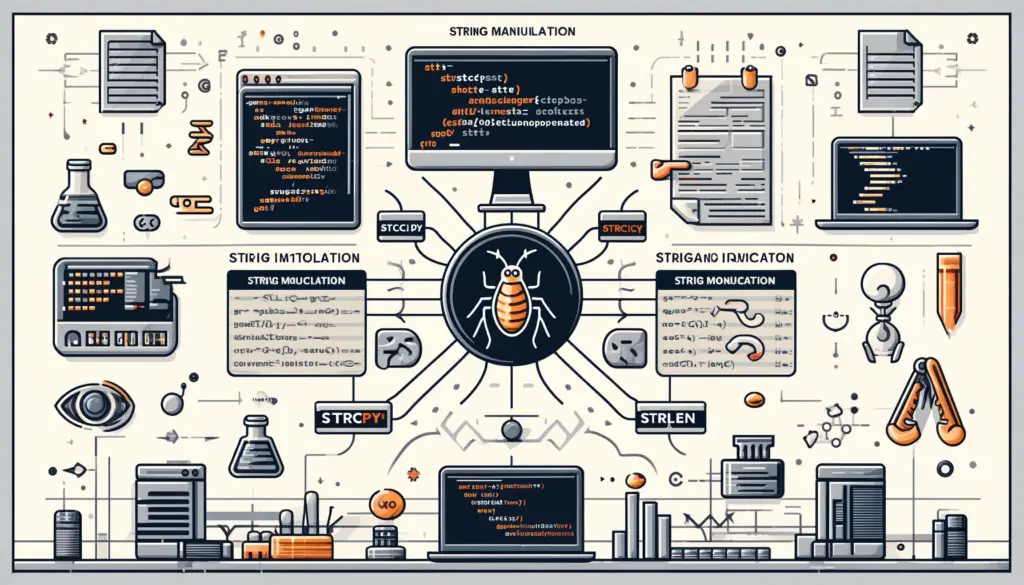
strcpy
は、ある文字列を別の文字列にコピーするために使用します。
基本構文:
char *strcpy(char *destination, const char *source);
例:文字列をコピーするプログラム
#include <stdio.h>
#include <string.h>
int main() {
char source[] = "Hello, World!";
char destination[50];
strcpy(destination, source);
printf("Source: %s\n", source);
printf("Destination: %s\n", destination);
return 0;
}
解説:
strcpy
はsource
の内容をdestination
にコピーします。destination
のサイズがsource
より小さい場合、バッファオーバーフローのリスクがあります。
2. strcat関数:文字列の連結
strcat
は、1つの文字列の末尾に別の文字列を連結します。
基本構文:
char *strcat(char *destination, const char *source);
例:文字列を連結するプログラム
#include <stdio.h>
#include <string.h>
int main() {
char greeting[50] = "Hello, ";
char name[] = "Alice";
strcat(greeting, name);
printf("%s\n", greeting);
return 0;
}
解説:
strcat
はgreeting
の末尾にname
を追加します。- 連結先の配列サイズが十分であることを確認する必要があります。
3. strlen関数:文字列の長さを測定
strlen
は、文字列の長さ(ヌル文字'\\0'
を除く)を返します。
基本構文:
size_t strlen(const char *str);
例:文字列の長さを測定するプログラム
#include <stdio.h>
#include <string.h>
int main() {
char word[] = "C programming";
printf("Length of '%s': %lu\n", word, strlen(word));
return 0;
}
解説:
strlen
はヌル文字を含めずに文字列の長さを返します。- 配列全体のサイズを取得したい場合は
sizeof
を使用します。
4. 他の便利な文字列操作関数
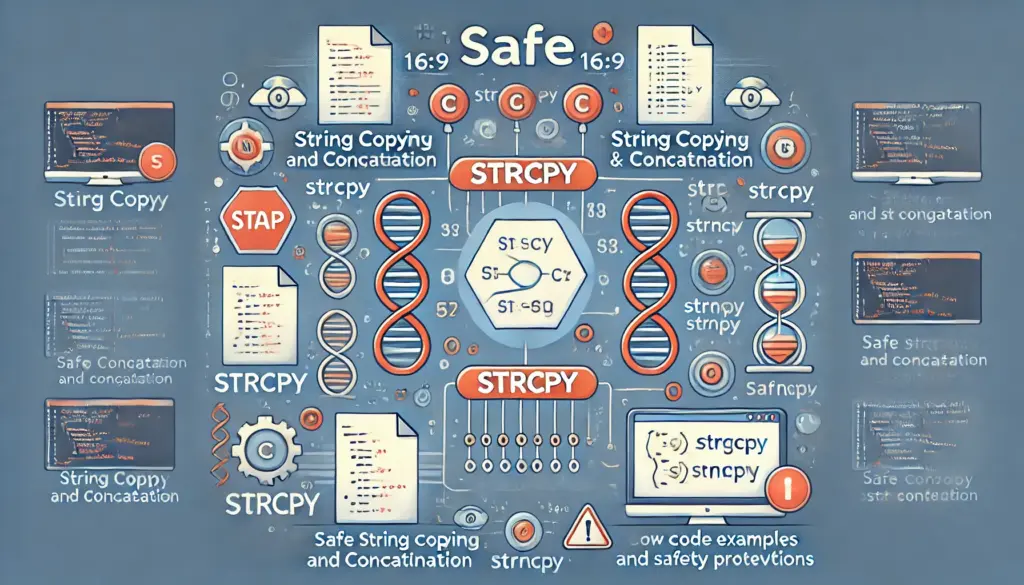
4.1 strcmp関数:文字列の比較
strcmp
は2つの文字列を辞書順で比較します。
int strcmp(const char *str1, const char *str2);
例:文字列を比較するプログラム
#include <stdio.h>
#include <string.h>
int main() {
char str1[] = "Apple";
char str2[] = "Banana";
int result = strcmp(str1, str2);
if (result < 0) {
printf("%s is less than %s\n", str1, str2);
} else if (result > 0) {
printf("%s is greater than %s\n", str1, str2);
} else {
printf("%s is equal to %s\n", str1, str2);
}
return 0;
}
解説:
strcmp
は文字列を比較し、str1
がstr2
より小さい場合は負の値、大きい場合は正の値、等しい場合は0を返します。
4.2 strncpy関数:安全な文字列コピー
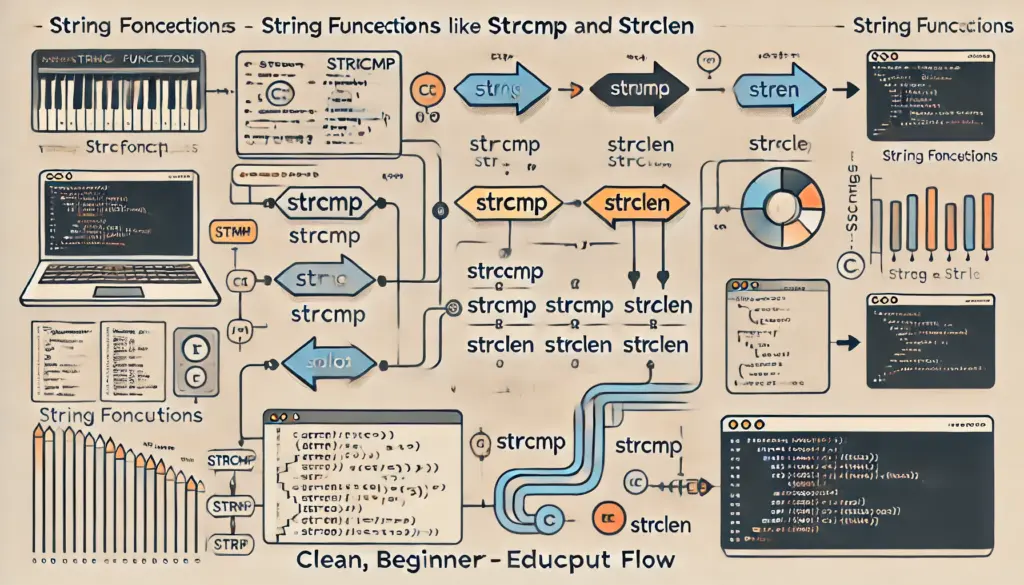
strncpy
は、指定した長さだけ文字列をコピーします。
#include <stdio.h>
#include <string.h>
int main() {
char source[] = "Hello, World!";
char destination[8];
strncpy(destination, source, 7); // 最初の7文字をコピー
destination[7] = '\\0'; // ヌル文字を追加
printf("Partial copy: %s\n", destination);
return 0;
}
解説:
strncpy
はコピーする文字数を指定できるため、安全性が高まります。- 手動でヌル文字を追加する必要がある場合があります。
5. 練習問題
以下の課題に挑戦して、文字列操作関数の使い方を練習しましょう。
- ユーザーから入力された2つの文字列を連結して出力するプログラムを作成してください。
- 文字列をコピーする際に、
strncpy
を使用して安全にコピーするプログラムを作成してください。 - 文字列を辞書順で比較して、結果を出力するプログラムを作成してください。
6. 練習問題の解答と解説
問1の解答
#include <stdio.h>
#include <string.h>
int main() {
char str1[50], str2[50];
printf("Enter first string: ");
scanf("%s", str1);
printf("Enter second string: ");
scanf("%s", str2);
strcat(str1, str2);
printf("Concatenated string: %s\n", str1);
return 0;
}
問2の解答
#include <stdio.h>
#include <string.h>
int main() {
char source[] = "Hello, World!";
char destination[8];
strncpy(destination, source, 7);
destination[7] = '\\0';
printf("Copied string: %s\n", destination);
return 0;
}
問3の解答
#include <stdio.h>
#include <string.h>
int main() {
char str1[50], str2[50];
printf("Enter first string: ");
scanf("%s", str1);
printf("Enter second string: ");
scanf("%s", str2);
int result = strcmp(str1, str2);
if (result < 0) {
printf("%s is less than %s\n", str1, str2);
} else if (result > 0) {
printf("%s is greater than %s\n", str1, str2);
} else {
printf("%s is equal to %s\n", str1, str2);
}
return 0;
}
7. まとめ
文字列操作関数を活用することで、効率的に文字列を操作できます。strcpy
やstrcat
、strlen
などを安全に使用するために、適切なサイズ管理を行いましょう。