【C言語】第4章第11回:関数ポインタの基本と使い方
関数ポインタは、プログラムの柔軟性と汎用性を向上させるための重要なツールです。この章では、関数ポインタの基本的な仕組みから実用例までを解説します。
1. 関数ポインタとは何か?
1.1 基本概念
関数ポインタは、関数のアドレスを格納するポインタです。通常のポインタと同様に、関数のメモリアドレスを操作できます。
1.2 関数ポインタの宣言
関数ポインタは以下の形式で宣言します:
return_type (*pointer_name)(parameter_types);
例:整数を受け取って整数を返す関数のポインタ
int (*funcPtr)(int);
2. 関数ポインタの基本的な使い方
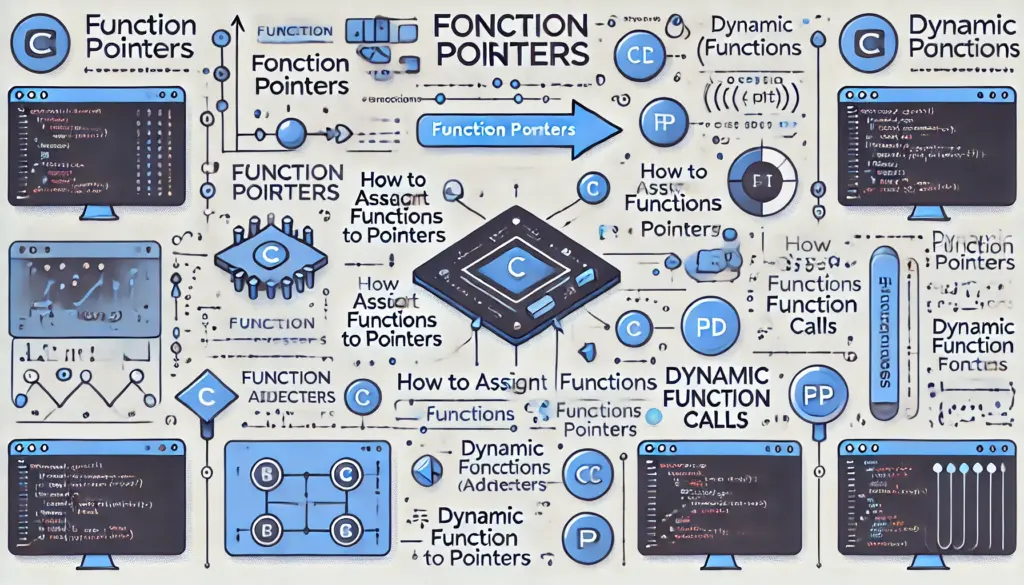
2.1 関数ポインタに関数を格納する
例:関数ポインタに関数アドレスを格納し呼び出す
#include <stdio.h>
int add(int a, int b) {
return a + b;
}
int main() {
int (*operation)(int, int); // 関数ポインタの宣言
operation = &add; // 関数アドレスを格納
printf("Result: %d\n", operation(5, 3)); // 関数呼び出し
return 0;
}
2.2 ポインタを使った関数呼び出し
関数ポインタを使うことで、動的に関数を切り替えられます。
例:複数の関数を動的に呼び出す
#include <stdio.h>
int add(int a, int b) { return a + b; }
int multiply(int a, int b) { return a * b; }
int main() {
int (*operations[])(int, int) = {add, multiply};
printf("Addition: %d\n", operations[0](5, 3));
printf("Multiplication: %d\n", operations[1](5, 3));
return 0;
}
3. 応用例:関数ポインタを使ったメニューシステム
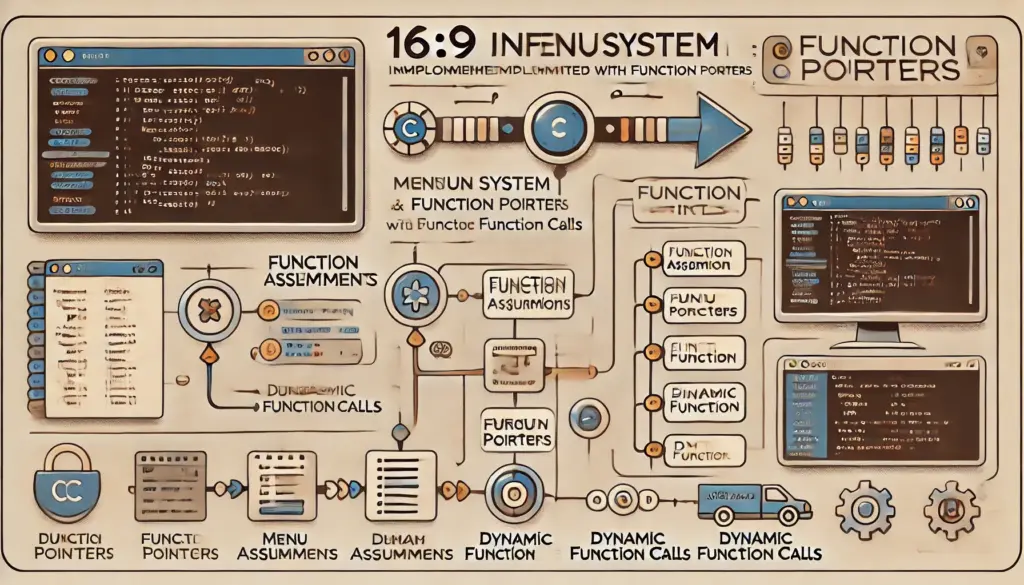
3.1 メニューシステムの設計
関数ポインタを使うと、動的なメニューシステムを構築できます。
3.2 実装例
例:関数ポインタを活用したシンプルなメニュー
#include <stdio.h>
void option1() { printf("Option 1 selected.\n"); }
void option2() { printf("Option 2 selected.\n"); }
void option3() { printf("Option 3 selected.\n"); }
int main() {
void (*menu[])(void) = {option1, option2, option3};
int choice;
printf("Menu:\n1. Option 1\n2. Option 2\n3. Option 3\nEnter your choice: ");
scanf("%d", &choice);
if (choice >= 1 && choice <= 3) {
menu[choice - 1]();
} else {
printf("Invalid choice.\n");
}
return 0;
}
4. 関数ポインタの安全な使い方
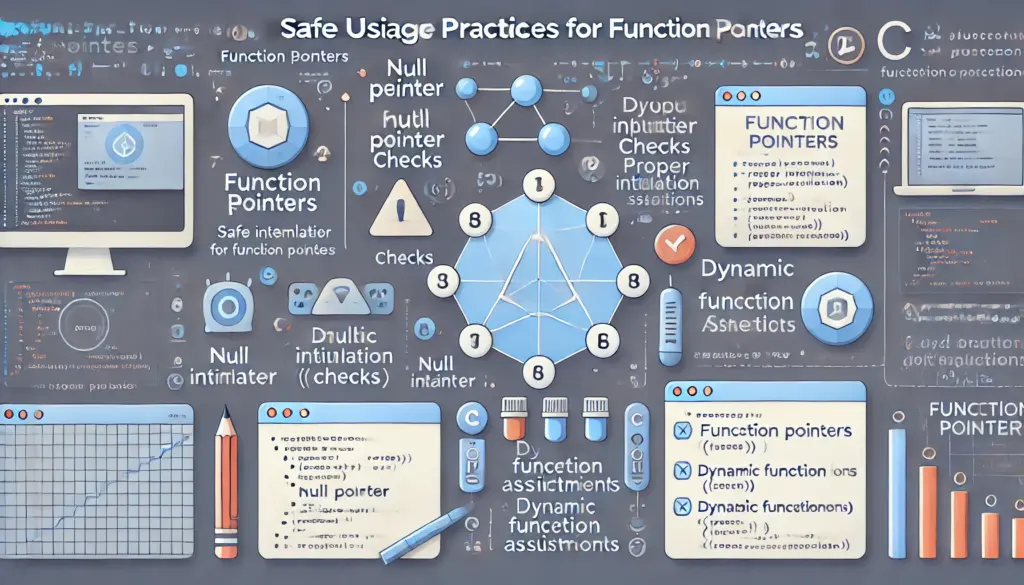
4.1 NULLチェック
関数ポインタがNULL
でないことを確認してから使用します。
例:NULLチェックの実装
#include <stdio.h>
void greet() {
printf("Hello, World!\n");
}
int main() {
void (*funcPtr)() = NULL; // 初期化
funcPtr = &greet;
if (funcPtr != NULL) {
funcPtr();
} else {
printf("Function pointer is NULL.\n");
}
return 0;
}
5. 練習問題
以下の課題に挑戦して、関数ポインタの使い方を深く理解しましょう。
- 関数ポインタを使って、複数の算術演算を動的に切り替えるプログラムを作成してください。
- 関数ポインタを配列として使用し、複数の関数を呼び出すメニューを作成してください。
- 関数ポインタを使って、指定された回数だけ文字列を出力する関数を呼び出すプログラムを作成してください。
6. 練習問題の解答と解説
問1の解答
#include <stdio.h>
int add(int a, int b) { return a + b; }
int subtract(int a, int b) { return a - b; }
int multiply(int a, int b) { return a * b; }
int main() {
int (*operations[])(int, int) = {add, subtract, multiply};
int choice, a = 5, b = 3;
printf("Choose operation:\n1. Add\n2. Subtract\n3. Multiply\n");
scanf("%d", &choice);
if (choice >= 1 && choice <= 3) {
printf("Result: %d\n", operations[choice - 1](a, b));
} else {
printf("Invalid choice.\n");
}
return 0;
}
7. まとめ
関数ポインタを使うことで、柔軟性の高いプログラムを構築できます。応用例を実践し、実際のプロジェクトで役立つスキルを磨きましょう。