【Python】第5章第15回:OOPを活用したプロジェクト例
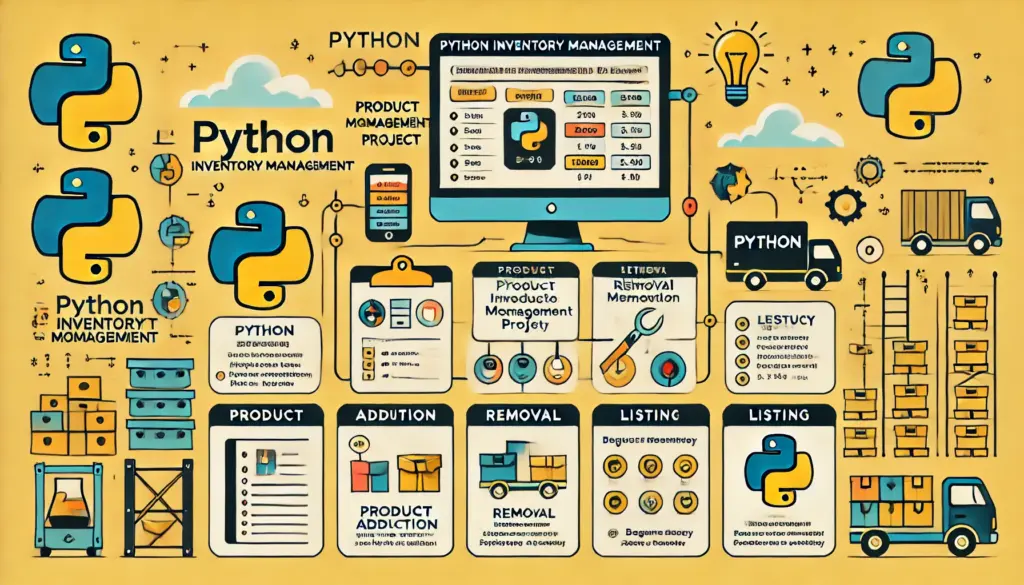
本記事では、オブジェクト指向プログラミング(OOP)を活用した実践的なプロジェクト例を解説します。PythonのOOPの概念をどのように活用するかを学ぶことで、プロジェクト全体の設計力を高めることができます。
0. 記事の概要
この記事を読むメリット
- OOPの応用力を向上:プロジェクトを通して実践的なスキルを身につけられます。
- 設計力の向上:クラス設計や継承の活用方法を深く理解できます。
- 保守性の高いコードの書き方を学ぶ:コードの再利用性や拡張性を向上させる技術を習得します。
この記事で学べること
- OOPを活用したプロジェクト設計の流れ
- クラス設計とその実装方法
- 設計を基にしたプロジェクトの実装と応用例
1. プロジェクト概要
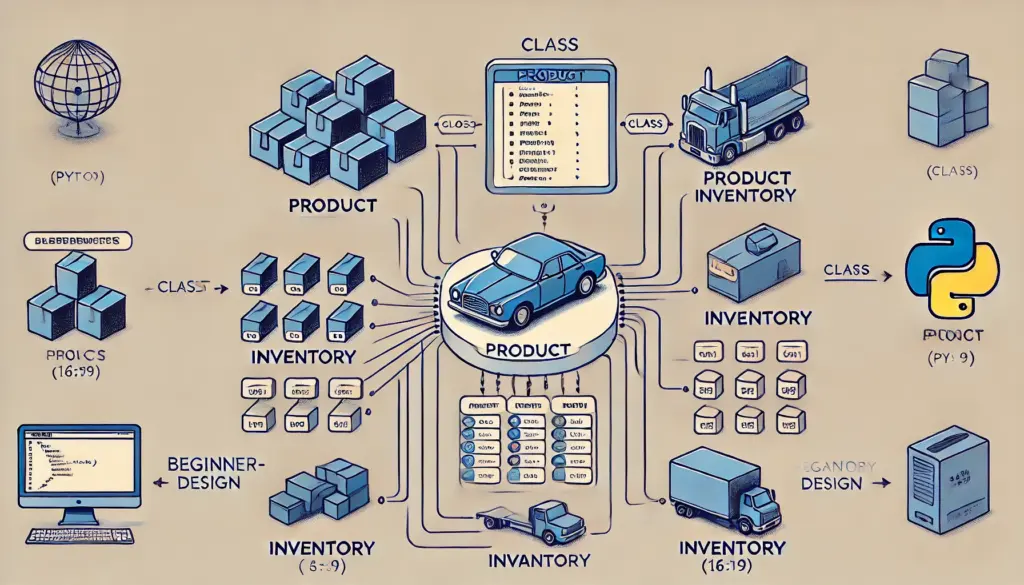
1.1 プロジェクトのテーマ
今回のプロジェクトでは、「シンプルな在庫管理システム」を構築します。OOPの特性を活かして、商品の管理、在庫の追加・削除、在庫一覧の表示を実現します。
1.2 必要な機能
- 商品を表すクラスの設計
- 在庫を管理するクラスの設計
- 在庫の追加・削除、在庫一覧表示のメソッド実装
2. 設計とクラス構造
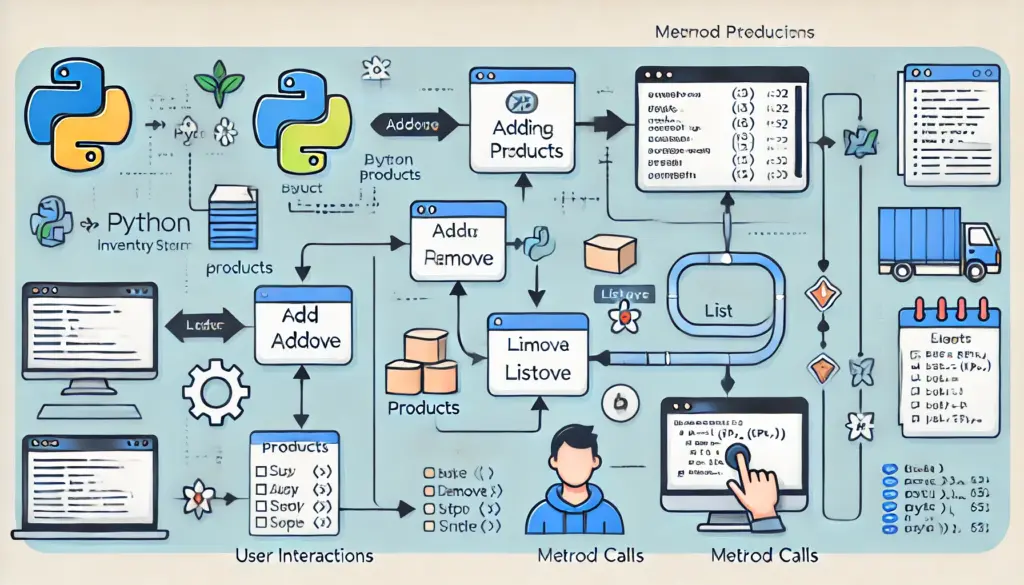
2.1 クラス図
以下のクラス図に基づいて設計を行います。
- Productクラス:商品の属性を表す。
- Inventoryクラス:商品の在庫を管理する。
2.2 クラスの実装例
# Productクラス
class Product:
def __init__(self, name, price, quantity):
self.name = name
self.price = price
self.quantity = quantity
def __str__(self):
return f"{self.name}: {self.price}円 ({self.quantity}個)"
# Inventoryクラス
class Inventory:
def __init__(self):
self.products = []
def add_product(self, product):
self.products.append(product)
def remove_product(self, product_name):
self.products = [p for p in self.products if p.name != product_name]
def list_products(self):
for product in self.products:
print(product)
# 使用例
inventory = Inventory()
product1 = Product("りんご", 100, 50)
product2 = Product("バナナ", 150, 30)
inventory.add_product(product1)
inventory.add_product(product2)
inventory.list_products()
動作解説
Product
クラスで商品の属性(名前、価格、数量)を管理します。Inventory
クラスで、商品の追加、削除、一覧表示を行います。- リストを使って複数の商品を管理しています。
3. プロジェクトの応用
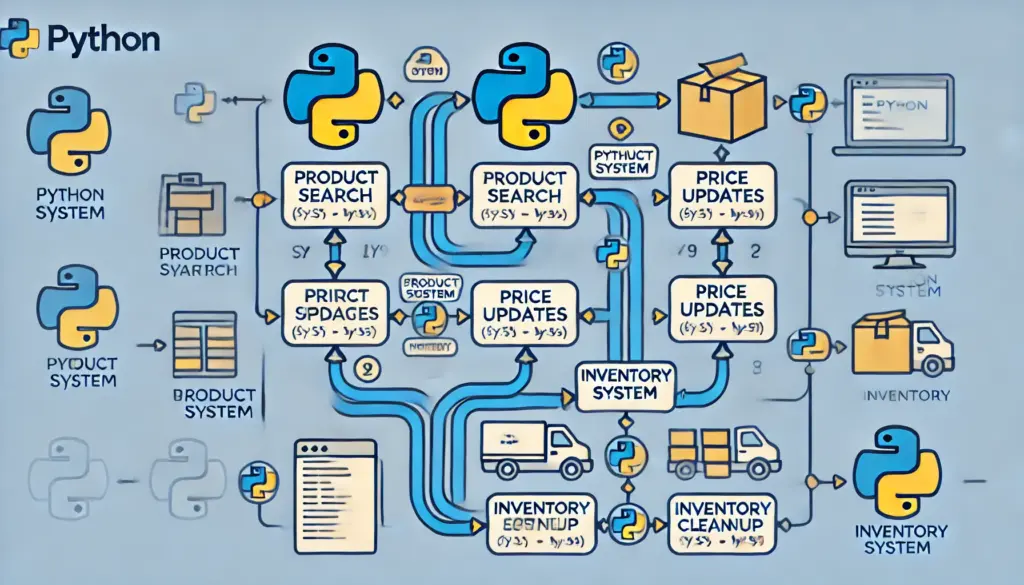
3.1 ユーザー入力を受け付けるシステム
# 簡易なユーザー入力システム
while True:
print("1. 商品追加 2. 商品削除 3. 商品一覧表示 4. 終了")
choice = input("選択してください: ")
if choice == "1":
name = input("商品名: ")
price = int(input("価格: "))
quantity = int(input("数量: "))
inventory.add_product(Product(name, price, quantity))
elif choice == "2":
name = input("削除する商品名: ")
inventory.remove_product(name)
elif choice == "3":
inventory.list_products()
elif choice == "4":
break
else:
print("無効な選択肢です。")
4. 練習問題
以下の課題に挑戦してみましょう。
- 商品の検索機能を追加してください。
- 商品の価格を変更する機能を追加してください。
- 在庫が0以下の商品をリストから自動的に削除する機能を追加してください。
5. 練習問題の解答と解説
問1〜3の解答例
# 商品検索機能
def search_product(self, product_name):
for product in self.products:
if product.name == product_name:
return product
return None
# 価格変更機能
def update_price(self, product_name, new_price):
product = self.search_product(product_name)
if product:
product.price = new_price
# 在庫0以下の商品を削除
def clean_inventory(self):
self.products = [p for p in self.products if p.quantity > 0]
6. まとめ
本記事では、OOPを活用してシンプルな在庫管理システムを構築しました。これを応用することで、より複雑なシステムの設計や開発にも挑戦できます。